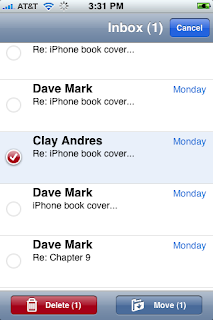
If you've played around at all with UITableView's "Edit Mode", you've probably been disappointed that it doesn't support the ability to select and then delete multiple rows in the table, the way that you can do in Apple's Mail application. It was one of the most welcome improvements made in the 2.0 iPhone OS, and I was a bit bummed when I discovered that the ability to do that was not being added to UITableView with the 2.0 release.
Personally, I'd like to see the functionality in more table-based iPhone apps, so I threw together a little sample iPhone project that shows how to do it. You can find the project Right Here.
I'm not going to walk through the entire projects, as most of it is standard application-building, but I wanted to point out the general approach I used. I do not know if this is exactly how Mail does it, and I'm certainly not sure this is the best way to do it, but it does work. The only aspect of the Mail implementation I didn't get is changing the background color of the selected rows in edit mode. I tried it, but was getting some weird behavior where the last-selected row was turning back to white, but when another row was added to the selection, then it would return to the correct color. At some point, I'll dive in and try and figure out what was wrong with that code, but for the time being, this should work pretty well for you and at least give you an idea of how the process can work.
In my controller class, I defined a few constants and macros:
#define kCellImageViewTag 1000
#define kCellLabelTag 1001
#define kLabelIndentedRect CGRectMake(40.0, 12.0, 275.0, 20.0)
#define kLabelRect CGRectMake(15.0, 12.0, 275.0, 20.0)
The first two will be used later to retrieve the correct subviews of the table view cell. The bottom two define the possible positions of the row's text. If we're in edit mode, the text is going to be moved over a little bit (which will be animated). By defining the two rects here, we can shift the text over easily by simply assigning the new value to the label's frame property.
In my controller, I have two mutable arrays. One will hold the display values, the other will be used to hold which rows are selected when we're in edit mode. I also define a BOOL that will identify when we're in edit mode. I don't call it edit mode because I don't want to risk a name conflict or confusion with Apple's code.
NSMutableArray *countries;
NSMutableArray *selectedArray;
BOOL inPseudoEditMode;
I also have two UIImage pointers that contain the checked and unchecked image. This is a little klugey - the unchecked is just a circle, so I probably should have just used CoreGraphics to draw the circle, but this was easier.
UIImage *selectedImage;
UIImage *unselectedImage;
I created a method that will create the selectedArray, populating it with NSNumber objects that hold a NO value for every row. This lets me easily reset the selection after a delete.
- (void)populateSelectedArray
{
NSMutableArray *array = [[NSMutableArray alloc] initWithCapacity:[countries count]];
for (int i=0; i < [countries count]; i++)
[array addObject:[NSNumber numberWithBool:NO]];
self.selectedArray = array;
[array release];
}
This method gets called in viewDidLoad, and also every time we delete rows. The viewDidLoad method also loads an array with strings pulled from a text file and loads the two UIImages.
There's an IBAction method to toggle edit mode. This gets called when the user presses the "Delete" button in the Nav Bar. It changes the value of inPsuedoEditMode and also hides or unhides the toolbar at the bottom, which has the "Delete" button that causes selected rows to get deleted.
-(IBAction)togglePseudoEditMode
{
self.inPseudoEditMode = !inPseudoEditMode;
toolbar.hidden = !inPseudoEditMode;
[self.tableView reloadData];
}
Most of the work here is in the tableView:cellForRowAtIndexPath: method. Here is where we have to look at whether we're in edit mode, and if we are in edit mode, look at which rows are selected.
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *EditCellIdentifier = @" editcell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:EditCellIdentifier];
if (cell == nil) {
cell = [[[UITableViewCell alloc] initWithFrame:CGRectZero reuseIdentifier:EditCellIdentifier] autorelease];
UILabel *label = [[UILabel alloc] initWithFrame:kLabelRect];
label.tag = kCellLabelTag;
[cell.contentView addSubview:label];
[label release];
UIImageView *imageView = [[UIImageView alloc] initWithImage:unselectedImage];
imageView.frame = CGRectMake(5.0, 10.0, 23.0, 23.0);
[cell.contentView addSubview:imageView];
imageView.hidden = !inPseudoEditMode;
imageView.tag = kCellImageViewTag;
[imageView release];
}
[UIView beginAnimations:@"cell shift" context:nil];
UILabel *label = (UILabel *)[cell.contentView viewWithTag:kCellLabelTag];
label.text = [countries objectAtIndex:[indexPath row]];
label.frame = (inPseudoEditMode) ? kLabelIndentedRect : kLabelRect;
UIImageView *imageView = (UIImageView *)[cell.contentView viewWithTag:kCellImageViewTag];
NSNumber *selected = [selectedArray objectAtIndex:[indexPath row]];
imageView.image = ([selected boolValue]) ? selectedImage : unselectedImage;
imageView.hidden = !inPseudoEditMode;
[UIView commitAnimations];
return cell;
}
Notice a few things
- we manually create subviews to the table view cell's content view, and we assign them tags. The tags allow us to retrieve the correct subview when we get dequeued cell instead of creating a new one.
- We call beginAnimations:forContext: and commitAnimations: around our changes so that the changes get animated for us. That's all we have to do to make turning edit mode on and off animated
When a row is touched, and we are in edit mode, we have to set the corresponding row in the selection array to YES if it's currently NO and vice versa.
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
[self.tableView deselectRowAtIndexPath:indexPath animated:YES];
if (inPseudoEditMode)
{
BOOL selected = [[selectedArray objectAtIndex:[indexPath row]] boolValue];
[selectedArray replaceObjectAtIndex:[indexPath row] withObject:[NSNumber numberWithBool:!selected]];
[self.tableView reloadData];
}
}
There's one more method that's key to this process, which is another action method that gets called when the Delete button in the toolbar gets pressed. Because this toolbar is only shown when we're in edit mode, we don't have to check that, we just do the delete. Because you can't delete objects from a collection while enumerating over them, this method is a little more complex than you might expect.
-(IBAction)doDelete
{
NSMutableArray *rowsToBeDeleted = [[NSMutableArray alloc] init];
NSMutableArray *indexPaths = [[NSMutableArray alloc] init];
int index = 0;
for (NSNumber *rowSelected in selectedArray)
{
if ([rowSelected boolValue])
{
[rowsToBeDeleted addObject:[countries objectAtIndex:index]];
NSUInteger pathSource[2] = {0, index};
NSIndexPath *path = [NSIndexPath indexPathWithIndexes:pathSource length:2];
[indexPaths addObject:path];
}
index++;
}
for (id value in rowsToBeDeleted)
{
[countries removeObject:value];
}
[self.tableView deleteRowsAtIndexPaths:indexPaths withRowAnimation:UITableViewRowAnimationFade];
[indexPaths release];
[rowsToBeDeleted release];
inPseudoEditMode = NO;
[self populateSelectedArray];
[self.tableView reloadData];
}
Anyway, I hope this is helpful to some people. If you have any questions, put them in the comments, or drop me an e-mail.
No comments:
Post a Comment