X Shear
| 1 0 0 |
|SHx 1 0 |
| 0 0 1 |
Y Shear
| 1 ShY 0 |
| 0 1 0 |
| 0 0 1 |
So... we can make our own CGAffineTransformShear() functions. Here we go, fasten your seatbelts:
CGAffineTransformMakeShear.h
#import <UIKit/UIKit.h>
#import <CoreGraphics/CoreGraphics.h>
CGAffineTransform CGAffineTransformMakeXShear(CGFloat proportion);
CGAffineTransform CGAffineTransformXShear(CGAffineTransform src, CGFloat proportion);
CGAffineTransform CGAffineTransformMakeYShear(CGFloat proportion);
CGAffineTransform CGAffineTransformYShear(CGAffineTransform src, CGFloat proportion);
CGAffineTransformMakeShear.m
#import "CGAffineTransformShear.h"
CGAffineTransform CGAffineTransformMakeXShear(CGFloat proportion)
{
return CGAffineTransformMake(1.0, 0.0, proportion, 1.0, 0.0, 0.0);
}
CGAffineTransform CGAffineTransformXShear(CGAffineTransform src, CGFloat proportion)
{
return CGAffineTransformConcat(src, CGAffineTransformMakeXShear(proportion));
}
CGAffineTransform CGAffineTransformMakeYShear(CGFloat proportion)
{
return CGAffineTransformMake(1.0, proportion, 0.0, 1.0, 0.0, 0.0);
}
CGAffineTransform CGAffineTransformYShear(CGAffineTransform src, CGFloat proportion)
{
return CGAffineTransformConcat(src, CGAffineTransformMakeYShear(proportion));
}
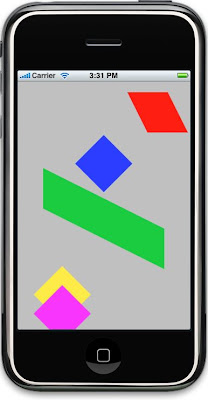
Now, we can shear a view, even one we create in Interface Builder:
The red and the green parallelograms in the screenshot to the left are just UIViews with a shear transform applied, using the functions above.
Have fun!
Oh, and if you want it - here's the revised Xcode project from the last article using shear.
No comments:
Post a Comment